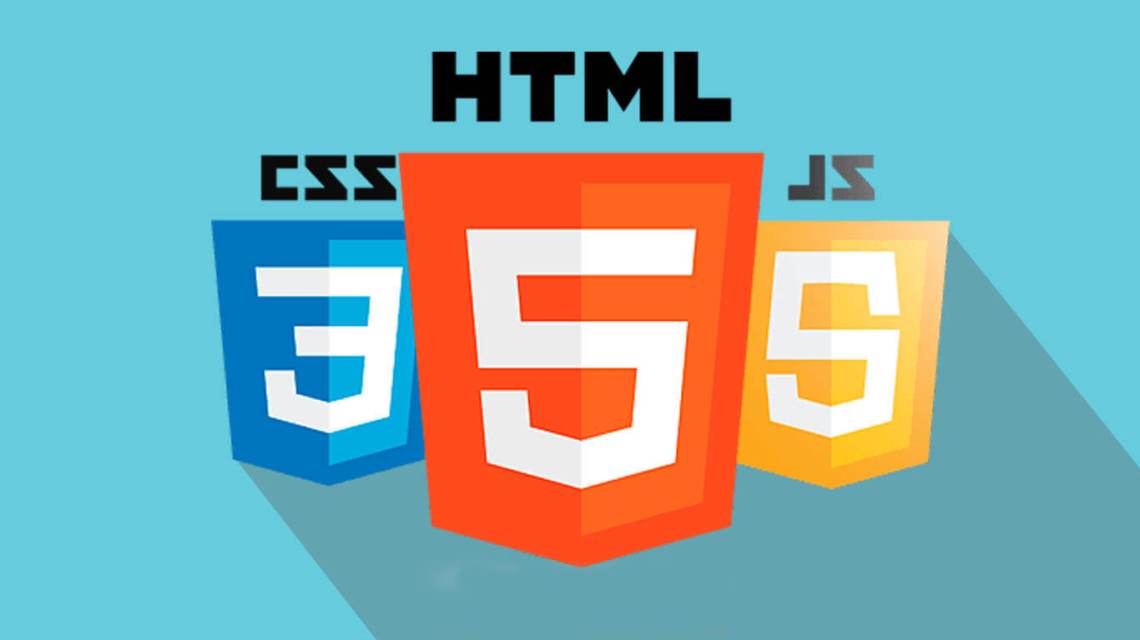
In the world of web development, creating interactive experiences has become a cornerstone of user engagement. In this code showcase, we’ll take you through the process of building an interactive web game using the trio of web technologies: HTML, CSS, and JavaScript. By the end of this tutorial, you’ll have a solid foundation for crafting your own web games that captivate users and keep them coming back for more.
Setting the Stage: The Game Concept
Before we dive into the code, let’s outline the game concept. Our game will be a classic memory card-matching game, where players flip over cards to find matching pairs. It’s a simple yet effective way to demonstrate the power of interactivity using web technologies.
Step 1: Structuring the HTML
To start, we’ll create the basic structure of our game using HTML. This includes the game board, cards, and any necessary elements. Here’s a snippet of the HTML structure:
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<link rel=”stylesheet” href=”styles.css”>
<title>Memory Game</title>
</head>
<body>
<div class=”game-board”>
<!– Cards will go here –>
</div>
<script src=”script.js”></script>
</body>
</html>
Next, let’s add some style to our game using CSS. We’ll create a grid layout for the cards and add animations to make the game more visually appealing. Here’s a snippet from our styles.css
file:
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
font-family: Arial, sans-serif;
}
.game-board {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
max-width: 400px;
margin: 0 auto;
}
.card {
width: 100%;
height: 100px;
background-color: #fff;
border-radius: 5px;
display: flex;
align-items: center;
justify-content: center;
cursor: pointer;
font-weight: bold;
font-size: 18px;
transition: transform 0.3s ease;
}
Step 3: Adding Interactivity with JavaScript
Now comes the heart of our game – JavaScript! We’ll add interactivity by defining the game logic and user interactions. Here’s a snippet from our script.js
file:
const cards = document.querySelectorAll(‘.card’);
let hasFlippedCard = false;
let lockBoard = false;
let firstCard, secondCard;
function flipCard() {
if (lockBoard) return;
if (this === firstCard) return;
this.classList.add(‘flip’);
if (!hasFlippedCard) {
hasFlippedCard = true;
firstCard = this;
return;
}
secondCard = this;
checkForMatch();
}
function checkForMatch() {
let isMatch = firstCard.dataset.framework === secondCard.dataset.framework;
isMatch ? disableCards() : unflipCards();
}
function disableCards() {
firstCard.removeEventListener(‘click’, flipCard);
secondCard.removeEventListener(‘click’, flipCard);
}
function unflipCards() {
lockBoard = true;
setTimeout(() => {
firstCard.classList.remove(‘flip’);
secondCard.classList.remove(‘flip’);
resetBoard();
}, 1000);
}
function resetBoard() {
[hasFlippedCard, lockBoard] = [false, false];
[firstCard, secondCard] = [null, null];
}
cards.forEach(card => card.addEventListener(‘click’, flipCard));
Conclusion
Congratulations! You’ve just created an interactive web game using HTML, CSS, and JavaScript. This code showcase demonstrates the power of these technologies in building engaging user experiences. From structuring the HTML to adding interactivity with JavaScript, you’ve embarked on a journey that can lead to even more complex and captivating games. Feel free to expand upon this foundation and experiment with different game mechanics to create your own unique web games.
Remember, practice makes perfect, so keep coding and exploring the limitless possibilities of web development!